场景
如果我们定义了某个函数:
1function getPhone(size, type, screen, price=100) {
2 ...
3}
如果这个函数很稳定那没什么问题,但如果经常变动,比如新增参数。
1function getPhone(size, type, screen, price=100, discount) {
2 ...
3}
此时我们如果想继续使用 price
的默认值,调用的时候还必须显性的传 undefined
,getPhone(4.3, 'iOS', 'OLED', undefined, 0.8)
。
如果再增加一个带默认值的参数,就会看起来越来越怪。
1function getPhone(size, type, screen, price=100, discount, mode='test') {
2 ...
3}
如果这个函数在很多地方都调用过,改的时候还需要保证修改后其他地方传参是正常的。
此时可以借助建造者模式的思想去改造它。
建造者模式
看下 维基百科 给的定义:
The builder pattern is a design pattern designed to provide a flexible solution to various object creation problems in object-oriented programming. The intent of the Builder design pattern is to separate the construction of a complex object from its representation. It is one of the Gang of Four design patterns.
建造者模式属于创建型设计模式,也就是为了生成对象。它将复杂的创建过程从构造函数分离出来,然后就可以在不改变原有构造函数的基础上,创建各种各样的对象。
GoF
书中提供的做法就是新创建一个 Builder
类,对象的创建委托给 Builder
类,原始的类不做操作,只负责调用即可。
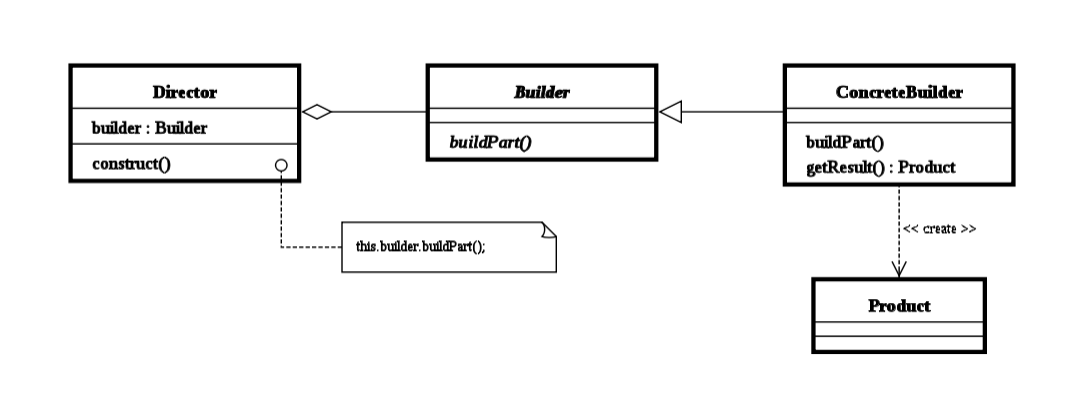
Director
类在构造函数中持有一个 Builder
实例,然后调用 Builder
类的 buildPart
和 getResult
即可创建对象。未来有新的对象需要创建的话,只需要实现新的 Builder
类即可,无需修改 Director
实例。
原始的建造者模式把对象的创建完全抽离到了 Builder
类中,这可能会导致原始类没啥用了,也许我们可以不全部抽离,Builder
类只负责接收参数即可。
以下示例来自极客时间的 设计模式之美
1public class ResourcePoolConfig {
2 private static final int DEFAULT_MAX_TOTAL = 8;
3 private static final int DEFAULT_MAX_IDLE = 8;
4 private static final int DEFAULT_MIN_IDLE = 0;
5
6 private String name;
7 private int maxTotal = DEFAULT_MAX_TOTAL;
8 private int maxIdle = DEFAULT_MAX_IDLE;
9 private int minIdle = DEFAULT_MIN_IDLE;
10
11 public ResourcePoolConfig(String name, Integer maxTotal, Integer maxIdle, Integer minIdle) {
12 if (StringUtils.isBlank(name)) {
13 throw new IllegalArgumentException("name should not be empty.");
14 }
15 this.name = name;
16
17 if (maxTotal != null) {
18 if (maxTotal <= 0) {
19 throw new IllegalArgumentException("maxTotal should be positive.");
20 }
21 this.maxTotal = maxTotal;
22 }
23
24 if (maxIdle != null) {
25 if (maxIdle < 0) {
26 throw new IllegalArgumentException("maxIdle should not be negative.");
27 }
28 this.maxIdle = maxIdle;
29 }
30
31 if (minIdle != null) {
32 if (minIdle < 0) {
33 throw new IllegalArgumentException("minIdle should not be negative.");
34 }
35 this.minIdle = minIdle;
36 }
37 }
38 //...省略getter方法...
39}
上边的 ResourcePoolConfig
类构造函数需要 4
个参数,如果经常变动,未来可能会越来越多,代码的可读性和易用性都会变差。因此这里可以用到建造者模式,但这里的建造者模式只用来传递参数,其他的逻辑还是维持在 ResourcePoolConfig
类中不变。
1public class ResourcePoolConfig {
2 private String name;
3 private int maxTotal;
4 private int maxIdle;
5 private int minIdle;
6
7 private ResourcePoolConfig(Builder builder) {
8 this.name = builder.name;
9 this.maxTotal = builder.maxTotal;
10 this.maxIdle = builder.maxIdle;
11 this.minIdle = builder.minIdle;
12 }
13 //...省略getter方法...
14
15 //我们将Builder类设计成了ResourcePoolConfig的内部类。
16 //我们也可以将Builder类设计成独立的非内部类ResourcePoolConfigBuilder。
17 public static class Builder {
18 private static final int DEFAULT_MAX_TOTAL = 8;
19 private static final int DEFAULT_MAX_IDLE = 8;
20 private static final int DEFAULT_MIN_IDLE = 0;
21
22 private String name;
23 private int maxTotal = DEFAULT_MAX_TOTAL;
24 private int maxIdle = DEFAULT_MAX_IDLE;
25 private int minIdle = DEFAULT_MIN_IDLE;
26
27 public ResourcePoolConfig build() {
28 // 校验逻辑放到这里来做,包括必填项校验、依赖关系校验、约束条件校验等
29 if (StringUtils.isBlank(name)) {
30 throw new IllegalArgumentException("...");
31 }
32 if (maxIdle > maxTotal) {
33 throw new IllegalArgumentException("...");
34 }
35 if (minIdle > maxTotal || minIdle > maxIdle) {
36 throw new IllegalArgumentException("...");
37 }
38
39 return new ResourcePoolConfig(this);
40 }
41
42 public Builder setName(String name) {
43 if (StringUtils.isBlank(name)) {
44 throw new IllegalArgumentException("...");
45 }
46 this.name = name;
47 return this;
48 }
49
50 public Builder setMaxTotal(int maxTotal) {
51 if (maxTotal <= 0) {
52 throw new IllegalArgumentException("...");
53 }
54 this.maxTotal = maxTotal;
55 return this;
56 }
57
58 public Builder setMaxIdle(int maxIdle) {
59 if (maxIdle < 0) {
60 throw new IllegalArgumentException("...");
61 }
62 this.maxIdle = maxIdle;
63 return this;
64 }
65
66 public Builder setMinIdle(int minIdle) {
67 if (minIdle < 0) {
68 throw new IllegalArgumentException("...");
69 }
70 this.minIdle = minIdle;
71 return this;
72 }
73 }
74}
75
76// 这段代码会抛出IllegalArgumentException,因为minIdle>maxIdle
77ResourcePoolConfig config = new ResourcePoolConfig.Builder()
78 .setName("dbconnectionpool")
79 .setMaxTotal(16)
80 .setMaxIdle(10)
81 .setMinIdle(12)
82 .build();
这样的话我们可以通过 ResourcePoolConfig.Builder()
来设置参数,将生成的参数对象传递给 ResourcePoolConfig
类的构造函数即可。
这里可以看作是变种的建造者模式,我们不是创建不同的 Builder
类来创建对象,而是给 Builder
类传递不同的参数来创建不同的对象。
代码实现
这里也只讨论变种的建造者模式。
在 js
中,我们同样可以照猫画虎的引入一个 Builer
类来接受参数,然后将创建参数对象传递给原始类。
但之所以在 Java
中引入新的 Builder
类是因为 Java
只能通过类来创建对象,但在 js
中我们是可以通过字面量来创建对象的,并且 ES6
还提供了对象的解构语法,会让我们使用起来更加简洁。
我们只需要将参数列表聚合为一个对象,然后通过解构取参数即可。
1function getPhone(size, type, screen, price = 100, discount) {
2 console.log('size', size)
3 console.log('type', type)
4 console.log('screen', screen)
5 console.log('price', price)
6 console.log('discount', discount)
7}
我们只需要改成:
1function getPhone({ size, type = 'iOS', screen = 'OLED', price = 100, discount } = {}) {
2 console.log('size', size)
3 console.log('type', type)
4 console.log('screen', screen)
5 console.log('price', price)
6 console.log('discount', discount)
7}
8
9getPhone({ size: 4, discount: 0.1, type: 'android' }) // 只需要传递需要的参数
上边的写法可以很方便的设置默认值,并且参数的顺序也不再重要,未来再扩展的时候也不需要太担心其他地方调用时候传参是否会引起问题。
注意一下参数列表中 {...} = {}
后边的大括号最好写一下,不然如果用户调用函数的时候什么都没有传,解构就会直接失败了。
1function getPhone({ size, type = 'iOS', screen = 'OLED', price = 100, discount }) {
2 console.log('size', size)
3 console.log('type', type)
4 console.log('screen', screen)
5 console.log('price', price)
6 console.log('discount', discount)
7}
8
9getPhone()
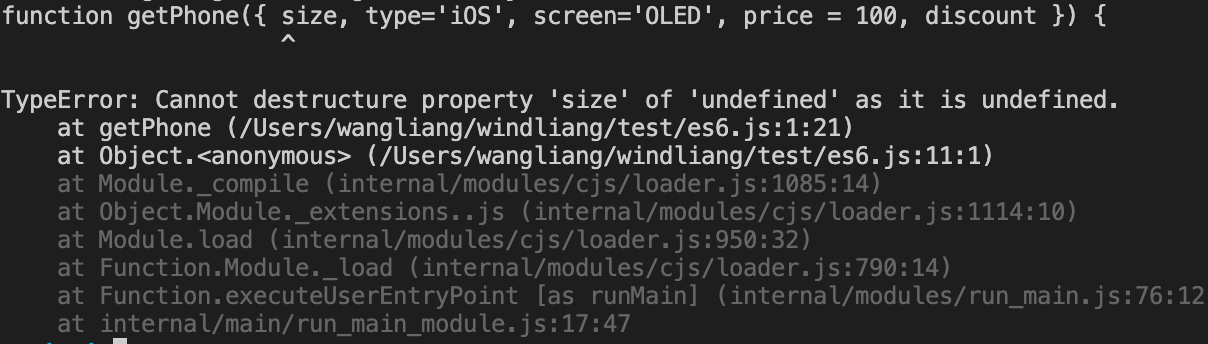
更多场景
通过对象来传递参数除了用在函数中以外,设计组件的时候,如果组件的参数会经常变动,并且越来越多,我们不妨引入一个 Object
类型的参数,然后将相关的参数内聚到 Object
中进行传递。
总
原始的建造者模式不清楚有没有实际应用,目前还没遇到,未来有的话再补充吧。
变种的建造者模式(只传递参数)在 js
中也很简单,直接通过对象传递参数即可。